python筆記12-python多線程之事件
前言
小夥伴a,b,c圍著吃火鍋,當菜上齊了,請客的主人說:開吃!,於是小夥伴一起動筷子,這種場景如何實現
一、 Event(事件)
Event(事件):事件處理的機制:全局定義了一個內置標誌Flag,如果Flag值為 False,那麼當程序執行 event.wait方法時就會阻塞,如果Flag值為True,那麼event.wait 方法時便不再阻塞。
Event其實就是一個簡化版的 Condition。Event沒有鎖,無法使線程進入同步阻塞狀態。
Event()
- set(): 將標誌設為True,並通知所有處於等待阻塞狀態的線程恢復運行狀態。
- clear(): 將標誌設為False。
- wait(timeout): 如果標誌為True將立即返回,否則阻塞線程至等待阻塞狀態,等待其他線程調用set()。
- isSet(): 獲取內置標誌狀態,返回True或False。
二、 Event案例1
場景:小夥伴a和b準備就緒,當收到通知event.set()的時候,會執行a和b線程
```
# coding:utf-8
import threading
import time
event = threading.Event()
def chihuoguo(name):
# 等待事件,進入等待阻塞狀態
print "%s 已經啟動" % threading.currentThread().getName()
print "小夥伴 %s 已經進入就餐狀態!"%name
time.sleep(1)
event.wait()
# 收到事件後進入運行狀態
print "%s 收到通知了." % threading.currentThread().getName()
print "小夥伴 %s 開始吃咯!"%name
# 設置線程組
threads = []
# 創建新線程
thread1 = threading.Thread(target=chihuoguo, args=("a", ))
thread2 = threading.Thread(target=chihuoguo, args=("b", ))
# 添加到線程組
threads.append(thread1)
threads.append(thread2)
# 開啟線程
for thread in threads:
thread.start()
time.sleep(0.1)
# 發送事件通知
print "主線程通知小夥伴開吃咯!"
event.set()
```
運行結果:
```
Thread-1 已經啟動
小夥伴 a 已經進入就餐狀態!
Thread-2 已經啟動
小夥伴 b 已經進入就餐狀態!
主線程通知小夥伴開吃咯!
Thread-1 收到通知了.
小夥伴 a 開始吃咯!
Thread-2 收到通知了.
小夥伴 b 開始吃咯!
```
二、 Event案例2
場景:當小夥伴a,b,c集結完畢後,請客的人發話:開吃咯!
```
# coding:utf-8
import threading
import time
event = threading.Event()
def chiHuoGuo(name):
# 等待事件,進入等待阻塞狀態
print "%s 已經啟動" % threading.currentThread().getName()
print "小夥伴 %s 已經進入就餐狀態!"%name
time.sleep(1)
event.wait()
# 收到事件後進入運行狀態
print "%s 收到通知了." % threading.currentThread().getName()
print "%s 小夥伴 %s 開始吃咯!"%(time.time(), name)
class myThread (threading.Thread): # 繼承父類threading.Thread
def __init__(self, people, name):
"""重寫threading.Thread初始化內容"""
threading.Thread.__init__(self)
self.threadName = name
self.people = people
def run(self): # 把要執行的代碼寫到run函數裡面 線程在創建後會直接運行run函數
"""重寫run方法"""
print("開始線程: " + self.threadName)
chiHuoGuo(self.people) # 執行任務
print("結束線程: " + self.name)
# 設置線程組
threads = []
# 創建新線程
thread1 = threading.Thread(target=chiHuoGuo, args=("a", ))
thread2 = threading.Thread(target=chiHuoGuo, args=("b", ))
thread3 = threading.Thread(target=chiHuoGuo, args=("c", ))
# 添加到線程組
threads.append(thread1)
threads.append(thread2)
threads.append(thread3)
# 開啟線程
for thread in threads:
thread.start()
time.sleep(0.1)
# 發送事件通知
print "YOYO:集合完畢,人員到齊了,開吃咯!"
event.set()
```
運行結果:
```
Thread-1 已經啟動
小夥伴 a 已經進入就餐狀態!
Thread-2 已經啟動
小夥伴 b 已經進入就餐狀態!
Thread-3 已經啟動
小夥伴 c 已經進入就餐狀態!
YOYO:集合完畢,人員到齊了,開吃咯!
Thread-1 收到通知了.
Thread-3 收到通知了.Thread-2 收到通知了.
```

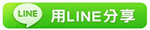
TAG:從零開始學自動化測試 |