Python-文件處理
文件通常用於存儲數據或應用系統的參數。Python提供了os、os.path、shutil等模塊用於處理文件,其中包括打開文件、讀寫文件、複製和刪除文件等函數。
文件的打開或創建
open(name[,mode[,buffering]])
name被打開的文件名
mode文件打開模式
buffering設置緩存模式。0表示不緩存,1表示表示行緩衝;如果大於1則表示緩衝區的大小,以位元組為單位。
open()返回一個file對象,file對象可對文件進行各種操作。
文件處理3步曲:
創建並打開文件,使用open()函數返回1個file對象
調用file()對象的read()、write()等方法處理文件
調用close()關閉文件,釋放file對象佔用的資源。
context ="""hello zero!
hello one!
hello two!
hello three!
hello four!
hello five!
hello six!
hello seven!
hello eight!
"""
#以讀寫入的方式創建一個文件
f=open("filetest.txt","w+",encoding="utf-8")
#向文件寫入內容context
f.write(context)
f.close()
#以只讀的的方式打開一個文件
f1=open("filetest.txt","r",encoding="utf-8")
print(f1.read())
f1.closed
out:
hello zero!
hello one!
hello two!
hello three!
hello four!
hello five!
hello six!
hello seven!
hello eight!
文件的讀取
read()一次性讀取文件內容
#以只讀的形式打開文件
f=open("filetest.txt","r",
encoding="utf-8")
#列印文件名
print("文件名:",f.name)
#一次性讀取文件內的所有內容
print("文件內容:
",f.read())
f.closed
out:
文件名: filetest.txt
文件內容
hello zero!
hello one!
hello two!
hello three!
hello four!
hello five!
hello six!
hello seven!
hello eight!
readline()逐行讀取文件內容
#以只讀的形式打開文件
f=open("filetest.txt","r",
encoding="utf-8")
while True:
line=f.readline()
ifline:
print(line)
else:
break
f.closed
out:
hello zero!
hello one!
hello two!
hello three!
hello four!
hello five!
hello six!
hello seven!
hello eight!
readlines()多行讀取文件內容
#以只讀的形式打開文件
f=open("filetest.txt","r",
encoding="utf-8")
lines= f.readlines()
forlineinlines:
print(line)
f.closed
out:
hello zero!
hello one!
hello two!
hello three!
hello four!
hello five!
hello six!
hello seven!
hello eight!
文件的寫入
context ="""hello zero!
hello one!
hello two!
hello three!
hello four!
hello five!
hello six!
hello seven!
hello eight!
"""
seq= ["1
","2
","3
","4
",
"5
","6
","7
","8
"]
#以讀寫入的方式打開或創建一個文件
f=open("filetest.txt","w+",
encoding="utf-8")
#向文件寫入內容context
f.write(context)
#向文件寫入字元串序列seq
f.writelines(seq)
#關閉文件
f.close()
#檢查是否寫入成功
f1=open("filetest.txt","r",
encoding="utf-8")
print(f1.read())
f1.closed
out:
hello zero!
hello one!
hello two!
hello three!
hello four!
hello five!
hello six!
hello seven!
hello eight!
1
2
3
4
5
6
7
8
文件的刪除
文件的刪除需要使用os模塊。os模塊提供了對系統環境、文件、目錄等操作系統級的介面函數。
文件的複製
file類沒有提供直接複製文件的方法,但是可以使用read()、write()方法模擬文件拷貝的功能。
seq= ["1
","2
","3
","4
",
"5
","6
","7
","8
"]
#以讀寫入的方式打開或創建一個文件
f=open("filetest.txt","w+",
encoding="utf-8")
#向文件寫入內容context
f.writelines(seq)
#關閉文件
f.close()
f=open("filetest.txt","r",
encoding="utf-8")
f1=open("filetest1.txt","w+",
encoding="utf-8")
#讀取文件f的所有內容
context=f.read()
f1.write(context)
f.close()
f1.close()
#檢查的文件內容
f1=open("filetest1.txt","r",
encoding="utf-8")
print(f1.read())
f1.close()
out:
1
2
3
4
5
6
7
8
shutil模塊實現文件的複製
importshutil
importos
ifos.path.exists(r"E:python text
filetest.txt"):
print("filetest文件存在")
else:
print("filetest文件不存在")
ifos.path.exists(r"E:python text
filetest4.txt"):
print("filetest4文件存在")
else:
print("filetest4文件不存在")
shutil.copyfile("filetest.txt","
filetest4.txt")
f4=open("filetest4","r")
print(f4.read())
f4.close()
#獲取f4所在路徑
print(os.path.abspath("filetest4.txt』)
文件的剪切
文件的重命名
importos
seq= ["1
","2
","3
","4
",
"5
","6
","7
","8
"]
#以讀寫入的方式打開或創建一個文件
f=open("filetest.txt","w+",encoding="utf-8")
#向文件寫入內容context
f.writelines(seq)
#關閉文件
f.close()
ifos.path.exists("filetest.txt"):
print("filetest.txt文件存在")
#重命名
os.rename("filetest.txt","file.txt")
ifos.path.exists("filetest.txt"):
print("filetest.txt文件存在")
ifos.path.exists("file.txt"):
print("file.txt文件存在")
out:
filetest.txt文件存在
file.txt文件存在
按類型查找文件
importglob
importos
print(glob.glob(r".*.txt"))
print(glob.glob(r".*.png"))
out:
[".\cookie.txt",".\f-1.txt",".\file.txt",
".\imooc.txt",".\text.txt"]
[".\3c07731b62dda41272ecacb65a414175.png",".\fig.png",
".\python總體架構.png"]
文件內容的查找和替換
文件內容的查找和替換,實際上是轉換為單個字元串的查找和替換
文件內容的查找
importre
f=open("file.txt","r")
f.seek(,)
count =
lines=f.readlines()
forlineinlines:
li = re.findall(r"8",line)
iflen(li)>:
count=count+li.count("8")
print("查找到"+str(count)+"個8")
文件內容的替換
f=open("file.txt","r")
f1=open("file2.txt","w")
lines=f.readlines()
forlineinlines:
f1.write(line.replace("8","6"))
f.close()
f1.close()
f1=open("file2.txt","r")
print(f1.read())
f1.close()
out:
16
26
36
46
56
66
76
66666666666666666
文件內容的比較
模塊difflib可用於實現對序列、文件的比較。
importdifflib
f=open("file.txt","r")
f1=open("file2.txt","r")
f_context=f.read()
f1_context=f1.read()
print(f_context)
print(f1_context)
#lambda x:x==""表示忽略換行符
s=difflib.SequenceMatcher(lambdax:x=="",
f_context,f1_context)
fortag,i1,i2,j1,j2ins.get_opcodes():
print("%s f_context[%d:%d]=%sf1_context(%d:%d)=%s"%
(tag,i1,i2,f_context[i1:i2],j1,j2,f1_context[j1:j2]))
out:
18
28
38
48
58
68
78
88888888888888888
16
26
36
46
56
66
76
66666666666666666
equal f_context[:1]=1f1_context(:1)=1
replace f_context[1:2]=8f1_context(1:2)=6
equal f_context[2:4]=
2f1_context(2:4)=
2
replace f_context[4:5]=8f1_context(4:5)=6
equal f_context[5:7]=
3f1_context(5:7)=
3
replace f_context[7:8]=8f1_context(7:8)=6
equal f_context[8:10]=
4f1_context(8:10)=
4
replace f_context[10:11]=8f1_context(10:11)=6
equal f_context[11:13]=
5f1_context(11:13)=
5
replace f_context[13:14]=8f1_context(13:14)=6
equal f_context[14:16]=
6f1_context(14:16)=
6
replace f_context[16:17]=8f1_context(16:17)=6
equal f_context[17:19]=
7f1_context(17:19)=
7
replace f_context[19:20]=8f1_context(19:20)=6
equal f_context[20:21]=
f1_context(20:21)=
replace f_context[21:38]=88888888888888888
f1_context(21:38)=66666666666666666
打開模式參數表
file類常用屬性和方法

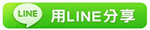
※簡單定義Python和Scala的類和對象
※你都用Python做過哪些騷操作?
TAG:Python |