AOP實現日誌記錄(Aspect)
2018年01月08日 12:06:44
閱讀數:106
//@Aspect用到的jar包
<!--aop日誌 -->
<dependency>
<groupId>org.aspectj</groupId>
<artifactId>aspectjweaver</artifactId>
<version>1.7.2</version>
</dependency>
//本人沒用到XML配置文件,所以用的是此註解 @EnableAspectJAutoProxy ,意思是啟用自動代理功能。次註解主要加在配置類里,如下圖,如果用的XML配置文件,只需要再XML里配置切點,切面即可(此處不做說明)
/**
*自定義註解 攔截Controller
*/
@Target({ElementType.PARAMETER, ElementType.METHOD})
@Retention(RetentionPolicy.RUNTIME)
@Documented
public @interface SystemControllerLog {
String description() default "";
}
import com.google.gson.Gson;
import org.apache.commons.lang3.StringUtils;
import org.aspectj.lang.JoinPoint;
import org.aspectj.lang.ProceedingJoinPoint;
import org.aspectj.lang.annotation.*;
import org.aspectj.lang.reflect.MethodSignature;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.stereotype.Component;
import org.springframework.web.context.request.RequestAttributes;
import org.springframework.web.context.request.RequestContextHolder;
import org.springframework.web.context.request.ServletRequestAttributes;
import javax.servlet.http.HttpServletRequest;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.lang.reflect.Method;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.HashMap;
import java.util.Map;
/**
*
* @ClassName: LogAspect
* @Description: 日誌記錄AOP實現
* @author shaojian.yu
* @date 2014年11月3日 下午1:51:59
*
*/
@Aspect
@Component
public class LogAspect {
private final Logger logger = LoggerFactory.getLogger(this.getClass());
private String requestPath = null ; // 請求地址
private String userNo = null ; // 用戶名
private Map<?,?> inputParamMap = null ; // 傳入參數
private Map<String, Object> outputParamMap = null; // 存放輸出結果
private long startTimeMillis = 0; // 開始時間
private long endTimeMillis = 0; // 結束時間
private String remoteAddr; //請求IP
private String discription; //方法描述
/**
* Controller層切點 註解攔截
*/
@Pointcut("@annotation(com.zhuohuiclass.cloud.web.annotation.SystemControllerLog)")
public void controllerAspect(){
}
/**
*
* @Title:doBeforeInServiceLayer
* @Description: 方法調用前觸發
* 記錄開始時間
* @author shaojian.yu
* @date 2014年11月2日 下午4:45:53
* @param joinPoint
*/
@Before("execution(* com.zhuohuiclass.cloud.web.controllers..*.*(..))")
public void doBeforeInServiceLayer(JoinPoint joinPoint) {
startTimeMillis = System.currentTimeMillis(); // 記錄方法開始執行的時間
}
/**
*
* @Title:doAfterInServiceLayer
* @Description: 方法調用後觸發
* 記錄結束時間
* @author shaojian.yu
* @date 2014年11月2日 下午4:46:21
* @param joinPoint
*/
@After("controllerAspect()")
public void doAfterInServiceLayer(JoinPoint joinPoint) throws IOException {
endTimeMillis = System.currentTimeMillis(); // 記錄方法執行完成的時間
discription = getControllerMethodDescription2(joinPoint);
if(StringUtils.isBlank(discription)){
discription = "-";
}
this.printOptLog();
}
/**
*
* @Title:doAround
* @Description: 環繞觸發
* @author shaojian.yu
* @date 2014年11月3日 下午1:58:45
* @param pjp
* @return
* @throws Throwable
*/
@Around("execution(* com.zhuohuiclass.cloud.web.controllers..*.*(..))")
public Object doAround(ProceedingJoinPoint pjp) throws Throwable {
/**
* 1.獲取request信息
* 2.根據request獲取session
* 3.從session中取出登錄用戶信息
*/
RequestAttributes ra = RequestContextHolder.getRequestAttributes();
ServletRequestAttributes sra = (ServletRequestAttributes)ra;
HttpServletRequest request = sra.getRequest();
userNo = (String) request.getSession().getAttribute("user");
if(StringUtils.isBlank(userNo)){
userNo = "-";
}
remoteAddr = request.getRemoteAddr();//請求的IP
if(StringUtils.isBlank(remoteAddr)){
remoteAddr = "-";
}
// 獲取輸入參數
inputParamMap = request.getParameterMap();
// 獲取請求地址
requestPath = request.getRequestURI();
if(StringUtils.isBlank(requestPath)){
requestPath = "-";
}
// 執行完方法的返回值:調用proceed()方法,就會觸發切入點方法執行
outputParamMap = new HashMap<String, Object>();
Object result = pjp.proceed();// result的值就是被攔截方法的返回值
outputParamMap.put("result", result);
return result;
}
/**
* 獲取註解中對方法的描述信息 用於Controller層註解
*
* @param joinPoint 切點
* @return discription
*/
public static String getControllerMethodDescription2(JoinPoint joinPoint) {
MethodSignature signature = (MethodSignature) joinPoint.getSignature();
Method method = signature.getMethod();
SystemControllerLog controllerLog = method
.getAnnotation(SystemControllerLog.class);
String discription = controllerLog.description();
return discription;
}
/**
*
* @Title:printOptLog
* @Description: 輸出日誌
* @author shaojian.yu
* @date 2014年11月2日 下午4:47:09
*/
private void printOptLog() {
Gson gson = new Gson(); // 需要用到google的gson解析包
String optTime = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss").format(startTimeMillis);
String data1 = "用戶編號:"+userNo+"
"
+"請求地址:"+requestPath+"
"
+"請求IP:"+remoteAddr+"
"
+"方法描述:"+discription+"
"
+"開始時間:"+optTime+"
"
+"調用方法時間:"+(endTimeMillis - startTimeMillis)+"ms;"+"
"
+"參數:"+gson.toJson(inputParamMap)+"
"
+"返回參數:"+gson.toJson(outputParamMap)+"
";
logger.error(data1);
try {
Date now = new Date();
SimpleDateFormat sdf = new SimpleDateFormat("yyyyMMdd");
String logName = sdf.format(now);
//伺服器日誌地址 /data/website/zhuohuiclass/cloud/logs/
File logFile = new File("C:\Users\lenovo\Desktop", logName+".txt");
if (!logFile.exists()) {
//logFile.mkdirs();
logFile.createNewFile();
}
if(StringUtils.isNoneBlank()){
}
//數據分析用 用戶編號 IP 方法 時間
String data =userNo+" "+remoteAddr+" "+discription+" "+optTime+" "+"
";
FileOutputStream out = new FileOutputStream(logFile,true);
out.write(data.getBytes());
out.close();
}catch (Exception e){
}
}
//自定義註解使用方法

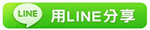
TAG:程序員小新人學習 |