跳一跳Python代碼
知識
07-12
- # coding: utf-8
- import os
- import sys
- import subprocess
- import shutil
- import time
- import math
- from PIL import Image, ImageDraw
- import random
- import json
- import re
- # === 思路 ===
- # 核心:每次落穩之後截圖,根據截圖算出棋子的坐標和下一個塊頂面的中點坐標,
- # 根據兩個點的距離乘以一個時間係數獲得長按的時間
- # 識別棋子:靠棋子的顏色來識別位置,通過截圖發現最下面一行大概是一條直線,就從上往下一行一行遍歷,
- # 比較顏色(顏色用了一個區間來比較)找到最下面的那一行的所有點,然後求個中點,
- # 求好之後再讓 Y 軸坐標減小棋子底盤的一半高度從而得到中心點的坐標
- # 識別棋盤:靠底色和方塊的色差來做,從分數之下的位置開始,一行一行掃描,由於圓形的塊最頂上是一條線,
- # 方形的上面大概是一個點,所以就用類似識別棋子的做法多識別了幾個點求中點,
- # 這時候得到了塊中點的 X 軸坐標,這時候假設現在棋子在當前塊的中心,
- # 根據一個通過截圖獲取的固定的角度來推出中點的 Y 坐標
- # 最後:根據兩點的坐標算距離乘以係數來獲取長按時間(似乎可以直接用 X 軸距離)
- # TODO: 解決定位偏移的問題
- # TODO: 看看兩個塊中心到中軸距離是否相同,如果是的話靠這個來判斷一下當前超前還是落後,便於矯正
- # TODO: 一些固定值根據截圖的具體大小計算
- # TODO: 直接用 X 軸距離簡化邏輯
- def open_accordant_config():
- screen_size = _get_screen_size()
- config_file = "{path}/config/{screen_size}/config.json".format(
- path=sys.path[0],
- screen_size=screen_size
- )
- if os.path.exists(config_file):
- with open(config_file, "r") as f:
- print("Load config file from {}".format(config_file))
- return json.load(f)
- else:
- with open("{}/config/default.json".format(sys.path[0]), "r") as f:
- print("Load default config")
- return json.load(f)
- def _get_screen_size():
- size_str = os.popen("adb shell wm size").read()
- m = re.search("(d+)x(d+)", size_str)
- if m:
- width = m.group(1)
- height = m.group(2)
- return "{height}x{width}".format(height=height, width=width)
- config = open_accordant_config()
- # Magic Number,不設置可能無法正常執行,請根據具體截圖從上到下按需設置
- under_game_score_y = config["under_game_score_y"]
- press_coefficient = config["press_coefficient"] # 長按的時間係數,請自己根據實際情況調節
- piece_base_height_1_2 = config["piece_base_height_1_2"] # 二分之一的棋子底座高度,可能要調節
- piece_body_width = config["piece_body_width"] # 棋子的寬度,比截圖中量到的稍微大一點比較安全,可能要調節
- # 模擬按壓的起始點坐標,需要自動重複遊戲請設置成「再來一局」的坐標
- if config.get("swipe"):
- swipe = config["swipe"]
- else:
- swipe = {}
- swipe["x1"], swipe["y1"], swipe["x2"], swipe["y2"] = 320, 410, 320, 410
- screenshot_backup_dir = "screenshot_backups/"
- if not os.path.isdir(screenshot_backup_dir):
- os.mkdir(screenshot_backup_dir)
- def pull_screenshot():
- process = subprocess.Popen("adb shell screencap -p", shell=True, stdout=subprocess.PIPE)
- screenshot = process.stdout.read()
- if sys.platform == "win32":
- screenshot = screenshot.replace(b"
", b"
") - f = open("autojump.png", "wb")
- f.write(screenshot)
- f.close()
- def backup_screenshot(ts):
- # 為了方便失敗的時候 debug
- if not os.path.isdir(screenshot_backup_dir):
- os.mkdir(screenshot_backup_dir)
- shutil.copy("autojump.png", "{}{}.png".format(screenshot_backup_dir, ts))
- def save_debug_creenshot(ts, im, piece_x, piece_y, board_x, board_y):
- draw = ImageDraw.Draw(im)
- # 對debug圖片加上詳細的注釋
- draw.line((piece_x, piece_y) + (board_x, board_y), fill=2, width=3)
- draw.line((piece_x, 0, piece_x, im.size[1]), fill=(255, 0, 0))
- draw.line((0, piece_y, im.size[0], piece_y), fill=(255, 0, 0))
- draw.line((board_x, 0, board_x, im.size[1]), fill=(0, 0, 255))
- draw.line((0, board_y, im.size[0], board_y), fill=(0, 0, 255))
- draw.ellipse((piece_x - 10, piece_y - 10, piece_x + 10, piece_y + 10), fill=(255, 0, 0))
- draw.ellipse((board_x - 10, board_y - 10, board_x + 10, board_y + 10), fill=(0, 0, 255))
- del draw
- im.save("{}{}_d.png".format(screenshot_backup_dir, ts))
- def set_button_position(im):
- # 將swipe設置為 `再來一局` 按鈕的位置
- global swipe_x1, swipe_y1, swipe_x2, swipe_y2
- w, h = im.size
- left = w / 2
- top = 1003 * (h / 1280.0) + 10
- swipe_x1, swipe_y1, swipe_x2, swipe_y2 = left, top, left, top
- def jump(distance):
- press_time = distance * press_coefficient
- press_time = max(press_time, 200) # 設置 200 ms 是最小的按壓時間
- press_time = int(press_time)
- cmd = "adb shell input swipe {x1} {y1} {x2} {y2} {duration}".format(
- x1=swipe["x1"],
- y1=swipe["y1"],
- x2=swipe["x2"],
- y2=swipe["y2"],
- duration=press_time
- )
- print(cmd)
- os.system(cmd)
- # 轉換色彩模式hsv2rgb
- def hsv2rgb(h, s, v):
- h = float(h)
- s = float(s)
- v = float(v)
- h60 = h / 60.0
- h60f = math.floor(h60)
- hi = int(h60f) % 6
- f = h60 - h60f
- p = v * (1 - s)
- q = v * (1 - f * s)
- t = v * (1 - (1 - f) * s)
- r, g, b = 0, 0, 0
- if hi == 0: r, g, b = v, t, p
- elif hi == 1: r, g, b = q, v, p
- elif hi == 2: r, g, b = p, v, t
- elif hi == 3: r, g, b = p, q, v
- elif hi == 4: r, g, b = t, p, v
- elif hi == 5: r, g, b = v, p, q
- r, g, b = int(r * 255), int(g * 255), int(b * 255)
- return r, g, b
- # 轉換色彩模式rgb2hsv
- def rgb2hsv(r, g, b):
- r, g, b = r/255.0, g/255.0, b/255.0
- mx = max(r, g, b)
- mn = min(r, g, b)
- df = mx-mn
- if mx == mn:
- h = 0
- elif mx == r:
- h = (60 * ((g-b)/df) + 360) % 360
- elif mx == g:
- h = (60 * ((b-r)/df) + 120) % 360
- elif mx == b:
- h = (60 * ((r-g)/df) + 240) % 360
- if mx == 0:
- s = 0
- else:
- s = df/mx
- v = mx
- return h, s, v
- def find_piece_and_board(im):
- w, h = im.size
- piece_x_sum = 0
- piece_x_c = 0
- piece_y_max = 0
- board_x = 0
- board_y = 0
- left_value = 0
- left_count = 0
- right_value = 0
- right_count = 0
- from_left_find_board_y = 0
- from_right_find_board_y = 0
- scan_x_border = int(w / 8) # 掃描棋子時的左右邊界
- scan_start_y = 0 # 掃描的起始y坐標
- im_pixel=im.load()
- # 以50px步長,嘗試探測scan_start_y
- for i in range(int(h / 3), int( h*2 /3 ), 50):
- last_pixel = im_pixel[0,i]
- for j in range(1, w):
- pixel=im_pixel[j,i]
- # 不是純色的線,則記錄scan_start_y的值,準備跳出循環
- if pixel[0] != last_pixel[0] or pixel[1] != last_pixel[1] or pixel[2] != last_pixel[2]:
- scan_start_y = i - 50
- break
- if scan_start_y:
- break
- print("scan_start_y: ", scan_start_y)
- # 從scan_start_y開始往下掃描,棋子應位於屏幕上半部分,這裡暫定不超過2/3
- for i in range(scan_start_y, int(h * 2 / 3)):
- for j in range(scan_x_border, w - scan_x_border): # 橫坐標方面也減少了一部分掃描開銷
- pixel = im_pixel[j,i]
- # 根據棋子的最低行的顏色判斷,找最後一行那些點的平均值,這個顏色這樣應該 OK,暫時不提出來
- if (50 < pixel[0] < 60) and (53 < pixel[1] < 63) and (95 < pixel[2] < 110):
- piece_x_sum += j
- piece_x_c += 1
- piece_y_max = max(i, piece_y_max)
- if not all((piece_x_sum, piece_x_c)):
- return 0, 0, 0, 0
- piece_x = piece_x_sum / piece_x_c
- piece_y = piece_y_max - piece_base_height_1_2 # 上移棋子底盤高度的一半
- for i in range(int(h / 3), int(h * 2 / 3)):
- last_pixel = im_pixel[0, i]
- # 計算陰影的RGB值,通過photoshop觀察,陰影部分其實就是背景色的明度V 乘以0.7的樣子
- h, s, v = rgb2hsv(last_pixel[0], last_pixel[1], last_pixel[2])
- r, g, b = hsv2rgb(h, s, v * 0.7)
- if from_left_find_board_y and from_right_find_board_y:
- break
- if not board_x:
- board_x_sum = 0
- board_x_c = 0
- for j in range(w):
- pixel = im_pixel[j,i]
- # 修掉腦袋比下一個小格子還高的情況的 bug
- if abs(j - piece_x) < piece_body_width:
- continue
- # 修掉圓頂的時候一條線導致的小 bug,這個顏色判斷應該 OK,暫時不提出來
- if abs(pixel[0] - last_pixel[0]) + abs(pixel[1] - last_pixel[1]) + abs(pixel[2] - last_pixel[2]) > 10:
- board_x_sum += j
- board_x_c += 1
- if board_x_sum:
- board_x = board_x_sum / board_x_c
- else:
- # 繼續往下查找,從左到右掃描,找到第一個與背景顏色不同的像素點,記錄位置
- # 當有連續3個相同的記錄時,表示發現了一條直線
- # 這條直線即為目標board的左邊緣
- # 然後當前的 y 值減 3 獲得左邊緣的第一個像素
- # 就是頂部的左邊頂點
- for j in range(w):
- pixel = im_pixel[j, i]
- # 修掉腦袋比下一個小格子還高的情況的 bug
- if abs(j - piece_x) < piece_body_width:
- continue
- if (abs(pixel[0] - last_pixel[0]) + abs(pixel[1] - last_pixel[1]) + abs(pixel[2] - last_pixel[2])
- > 10) and (abs(pixel[0] - r) + abs(pixel[1] - g) + abs(pixel[2] - b) > 10):
- if left_value == j:
- left_count = left_count+1
- else:
- left_value = j
- left_count = 1
- if left_count > 3:
- from_left_find_board_y = i - 3
- break
- # 邏輯跟上面類似,但是方向從右向左
- # 當有遮擋時,只會有一邊有遮擋
- # 算出來兩個必然有一個是對的
- for j in range(w)[::-1]:
- pixel = im_pixel[j, i]
- # 修掉腦袋比下一個小格子還高的情況的 bug
- if abs(j - piece_x) < piece_body_width:
- continue
- if (abs(pixel[0] - last_pixel[0]) + abs(pixel[1] - last_pixel[1]) + abs(pixel[2] - last_pixel[2])
- > 10) and (abs(pixel[0] - r) + abs(pixel[1] - g) + abs(pixel[2] - b) > 10):
- if right_value == j:
- right_count = left_count + 1
- else:
- right_value = j
- right_count = 1
- if right_count > 3:
- from_right_find_board_y = i - 3
- break
- # 如果頂部像素比較多,說明圖案近圓形,相應的求出來的值需要增大,這裡暫定增大頂部寬的三分之一
- if board_x_c > 5:
- from_left_find_board_y = from_left_find_board_y + board_x_c / 3
- from_right_find_board_y = from_right_find_board_y + board_x_c / 3
- # 按實際的角度來算,找到接近下一個 board 中心的坐標 這裡的角度應該是30°,值應該是tan 30°,math.sqrt(3) / 3
- board_y = piece_y - abs(board_x - piece_x) * math.sqrt(3) / 3
- # 從左從右取出兩個數據進行對比,選出來更接近原來老演算法的那個值
- if abs(board_y - from_left_find_board_y) > abs(from_right_find_board_y):
- new_board_y = from_right_find_board_y
- else:
- new_board_y = from_left_find_board_y
- if not all((board_x, board_y)):
- return 0, 0, 0, 0
- return piece_x, piece_y, board_x, new_board_y
- def dump_device_info():
- size_str = os.popen("adb shell wm size").read()
- device_str = os.popen("adb shell getprop ro.product.model").read()
- density_str = os.popen("adb shell wm density").read()
- print("如果你的腳本無法工作,上報issue時請copy如下信息:
********** -
Screen: {size}
Density: {dpi}
DeviceType: {type}
OS: {os}
Python: {python}
**********".format( - size=size_str.strip(),
- type=device_str.strip(),
- dpi=density_str.strip(),
- os=sys.platform,
- python=sys.version
- ))
- def check_adb():
- flag = os.system("adb devices")
- if flag == 1:
- print("請安裝ADB並配置環境變數")
- sys.exit()
- def main():
- h, s, v = rgb2hsv(201, 204, 214)
- print(h, s, v)
- r, g, b = hsv2rgb(h, s, v*0.7)
- print(r, g, b)
- dump_device_info()
- check_adb()
- while True:
- pull_screenshot()
- im = Image.open("./autojump.png")
- # 獲取棋子和 board 的位置
- piece_x, piece_y, board_x, board_y = find_piece_and_board(im)
- ts = int(time.time())
- print(ts, piece_x, piece_y, board_x, board_y)
- set_button_position(im)
- jump(math.sqrt((board_x - piece_x) ** 2 + (board_y - piece_y) ** 2))
- save_debug_creenshot(ts, im, piece_x, piece_y, board_x, board_y)
- backup_screenshot(ts)
- time.sleep(random.uniform(1.2, 1.4)) # 為了保證截圖的時候應落穩了,多延遲一會兒
- if __name__ == "__main__":
- main()

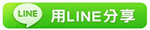
※IDEA 解決代碼提示功能消失
※scrollView滾動視圖實現商城模塊(附代碼)
TAG:程序員小新人學習 |