跳轉語句與數組基礎
首先繼續來談一下循環:while循環、do…while循環、for each循環。
while循環
與for循環一樣,while也是一個預測試的循環,但是while在循環開始之前,並不能確定重複執行循環開始語句序列的次數。while按不同的條件執行循環語句序列零次或多次。
例:求1+2+……的和,值之和大於1000為止。
package kkzz;
public class SumWhile {
public static void main(String[] args) {
int sum = 0;
int i = 0;
while (sum <= 10000){
sum += i;
i++;
}
System.out.printf("%d",sum);
}
}
1
2
3
4
5
6
7
8
9
10
11
12
10011
1
do…while循環
do語句按不同條件執行一次或多次循環語句序列。do…while循環的測試條件在執行完循環後執行,循環體至少執行一次,而while循環的循環體可能一次也不執行。
do…while循環語句的格式:
do{
循環體語句序列;
}while(條件表達式);
例:求1-100的和、奇數和、偶數和。
package com.k;
public class Dowhile {
public static int sum(int n) {
int sum = 0;
int i = 1;
do {
sum = sum + i;
i++;
} while (i <= n);//1-100求和
return sum;
}
public static int sumodd(int n) {
int sumOdd = 0;
int i = 1;
do {
sumOdd = sumOdd + i;
i += 2;
} while (i <= n);//1-100奇數求和
return sumOdd;
}
public static int sumeven(int n) {
int sumEven = 0;
int i = 2;
do {
sumEven = sumEven + i;
i += 2;
} while (i <= n);//1-100偶數求和
return sumEven;
}
public static void main(String[] args) {
System.out.println(sum(100));
System.out.println(sumodd(100));
System.out.println(sumeven(100));
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
5050
2500
2550
1
2
3
for each 語句
用於循環訪問數組或集合,以獲取所需信息。基本語句格式:for(變數:數據或集合){ 循環體語句序列;}
跳轉語句
跳轉語句用於無條件的轉移控制。使用跳轉語句執行分支,該語句會導致立即傳遞程序控制。Java提供了許多立即跳轉到程序中另一行代碼的語句,包括break、continue、return、throw。Java語言不支持goto語句。
break
break語句用於結束全部循環。
例:1+2+3+……n>1000時,輸出m 的值。
package com.k;
public class Dowhile {
public static int fun1(){
int i = 1;
int tmp = 0;
for(;tmp <= 1000;i ++){
tmp += i;
}
return i - 1;
} //只用for循環
public static int fun2(){
int i = 1;
int tmp = 0;
for(;;i ++){
tmp += i;
if(tmp > 1000){
break;
}
} //break 結束循環黨tmp大於1000時,返回i的值
return i;
}
public static void main(String[] args) {
System.out.println(fun1());
System.out.println(fun2());
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
45
45
1
2
continue
continue語句用於結束本次循環。
例:求既能被3整除又能被5整除的數字。
package com.k;
public class Dowhile {
ublic static void main(String[] args) {
System.out.println("1-100之間既能被3整除又能被5整除的數:");
for (int i = 1; i <= 100; i++) {
if (i % 15 != 0) {
continue;
}
System.out.println(i);
}
}
}
1
2
3
4
5
6
7
8
9
10
11
12
1-100之間既能被3整除又能被5整除的數:
15
30
45
60
75
90
1
2
3
4
5
6
7
return語句
return語句用於終止方法的執行並將控制返回給調用方法。如果方法有返回類型,return語句必須返回這個類型的值。如果方法為void類型,應使用沒有表達式的return語句;如果方法為void類型,方法體最後的return語句可以省略。
數組(Array)
數組是一種數據結構,包含相同類型的一組數據。數組本身是數組引用類型對象,數組元素可以是任何數據類型(簡單類型或引用類型),包括數組類型。
數組有一個「秩(rank)」,確定和每個數組元素的索引個數,其值是數組類型的方括弧對([])的個數。數組的秩又稱為數組的維度。「秩」為1的數組稱為一維數組,「秩」大於1的數組稱為多維數組。維度大小確定的多維數組通常稱為二維數組、三維數組等。
數組的每一個維度都有一個關聯的長度(length),它是一個大於或等於0的整數。創建數組實例時,將確定維度和各維度的長度,它們在該數組實例的整個生存期內保持不變。換言之,對於一個已存在的數組實例,既不能更改它的維度,也不能調整它的維度大小。
Java 語言中提供的數組是用來存儲固定大小的同類型元素。
數組的聲明:
首先必須聲明數組變數,才能在程序中使用數組。因為數組類型為引用類型,數組變數的聲明只是為數組實例的引用留出空間。數組的聲明有以下兩種形式:1.類型[] 數組變數名; //一般用該種形式; 2. 類型 數組變數名[];
例:
int[] arr1; //聲明一個整型數組
byte[] arrByte1; //聲明一個byte類型數組
1
2
數組的實例化(創建)和初始化:
數組在聲明後必須實例化才能使用。數組實例在運行時使用new運算符動態創建(即實例化)。
new運算符指定數組實例的長度。new運算符自動將數組的元素初始化為相應的默認值:簡單數值類型的數組元素默認值設置為零;char類型數組元素被初始化為0(u0000);boolean類型數組元素被初始化為false;而引用類型數組元素的默認值設置為null。使用new運算符創建數組時,還可以通過{}初始化數組的元素。
例:
int[] arr1; //聲明數組
arr1 = new int[10]; //創建一個可以存儲10個整數的數組(實例化數組)
int[] arr2 = new int[10]; //同時聲明和創建數組
arr2 = new int[]{1,2,3,4}; //創建並初始化一個可以存儲5個整數的數組
int[] arr3 = {1,2,3,4,5}; //聲明並初始化一個存儲4個整數的int數組(Java編譯器將自動出啊年數組)
String str[][] = new String[3][4]; //多維數組
1
2
3
4
5
6
如果通過{ }初始化數組的元素,則使用new運算符創建數組不需要也不能指定數組元素的個數,Java編譯器將自動推斷出數組的元素個數。 聲明數組時,可直接初始化(無需使用new運算符創建數組),Java編譯器將自動創建數組(實例化)。
數組的基本訪問操作
通過數組下標(或索引)來訪問數組中的數據元素。可以使用for each循環訪問數組的各個元素。數組下標從0開始,具有n個元素(即維度長度為n)的數組下標是 0~n-1。通過數組的length屬性,可以獲取數組的長度。注意:訪問數組時,如果使用了超過數組下標範圍的索引,即數組越界,則會引發ArrayIndexOutOfBoundsException。
例:
package kkee;
import java.util.Arrays;
public class NewArray {
public static void main(String[] args) {
int[] myList = {9, 2, 4, 5}; //聲明並初始化數組myList
// 列印所有數組元素
for (int i = 0; i < myList.length; i++) {
System.out.println(myList[i] + " ");
}
// 計算所有元素的總和
int total = 0;
for (int i = 0; i < myList.length; i++) {
total += myList[i];
}
System.out.println("Total is " + total);
// 查找最大元素
int max = myList[0];
for (int i = 1; i < myList.length; i++) {
if (myList[i] > max)
max = myList[i];
}
System.out.println("Max is " + max);
Arrays.sort(myList); //Arrays.sort 數組排序
System.out.println(Arrays.toString(myList));
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
9
2
4
5
Total is 20
Max is 9
[2, 4, 5, 9]
1
2
3
4
5
6
7
例:用數組列印斐波那契數列的前20項。
package com.k;
import java.util.Arrays; //導入Arrays包
public class Array {
public static void fabonacci(int[] array){ //數組作為函數的參數
int i;
array[0] = 1;
array[1] = 1;
for(i = 2;i < array.length; i++){
array[i] = array[i - 1] + array[i - 2];
}
}
public static void main(String[] args) {
int[] array = new int[20];
fabonacci(array);
System.out.println(Arrays.toString(array));
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
[1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89, 144, 233, 377, 610, 987, 1597, 2584, 4181, 6765]
1
例: for each輸出數組元素:
package kkee;
import java.util.Arrays;
/**
- @Package: kkee
- @Author:kkz
- @Description:
- @Date:Created in 2018/10/16 17:04
*/
public class Arrayforeach {
public static void main(String[] args) {
int[] array1 = new int[]{1,2,3,4,5};
for(int i = 0;i < array1.length;i++) {
System.out.print(array1[i] + " " );
}
System.out.println();
for(int i : array1) {
System.out.println(i);
}//for each 換行輸出數組元素
System.out.println(Arrays.toString(array1));//返回指定數組的字元串形式
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
1 2 3 4 5
1
2
3
4
5
[1, 2, 3, 4, 5]
1
2
3
4
5
6
7
Arrays 類
java.util.Arrays 類能方便地操作數組,它提供的所有方法都是靜態的。具有以下功能:
(1)給數組賦值:通過 fill 方法。
(2)對數組排序:通過 sort 方法,按升序。
(3)比較數組:通過 equals 方法比較數組中元素值是否相等。
(4)查找數組元素:通過 binarySearch 方法能對排序好的數組進行二分查找
法操作。
(5)複製數組:通過copyOf方法複製數組,截取或默認值填充,結果為相同數據類型的長度為指定長度的數組。
(6)複製數組的指定範圍內容:通過copyO分Range方法從起始索引(包括)到結束索引(不包括),結果為相同的數據類型的數組。
(7)返回指定數組內容的字元串形式:通過toString方法。
練習
1.實現二分查找演算法:有序的數組
pubclic static int binarySearch(int[] array,int key) {} 找到返回下標,沒有找到-1; 數組名,鍵值
二分查找演算法:
二分查找又稱折半查找,它是一種效率較高的查找方法。
折半查找的演算法思想是將數列按有序化(遞增或遞減)排列,查找過程中採用跳躍式方式查找,即先以有序數列的中點位置為比較對象,如果要找的元素值小 於該中點元素,則將待查序列縮小為左半部分,否則為右半部分。通過一次比較,將查找區間縮小一半。 折半查找是一種高效的查找方法。它可以明顯減少比較次數,提高查找效率。但是,折半查找的先決條件是查找表中的數據元素必須有序。
折半查找法的優點是比較次數少,查找速度快,平均性能好;其缺點是要求待查表為有序表,且插入刪除困難。因此,折半查找方法適用於不經常變動而查找頻繁的有序列表。
二分演算法步驟描述
① 首先確定整個查找區間的中間位置 middle;
② 用待查關鍵字值與中間位置的關鍵字值進行比較;
若相等,則查找成功
若大於,則在後(右)半個區域繼續進行折半查找
若小於,則在前(左)半個區域繼續進行折半查找
③ 對確定的縮小區域再按折半公式,重複上述步驟。
最後,得到結果:要麼查找成功, 要麼查找失敗。折半查找的存儲結構採用一維數組存放。
package mypratice;
/**
* @Package: mypratice
* @Author:kkz
* @Description:
* @Date:Created in 2018/10/16 17:15
*
*/
public class OrderArray {
public static void main(String[] args) {
int[] arr = {1, 2, 3, 4, 5, 6, 7, 8, 11, 15, 17};
int key = 15; //查找元素
System.out.println("所查找元素在數組中的下標為:" + binarySearch(arr, key, 0, 10));
}
public static int binarySearch(int[] arr, int key,int start,int end) {
start = 0;
end = arr.length - 1;
while (start <= end) {
int middle = start + (end - start) / 2;
if (arr[middle] == key) {
return middle;
} else if (arr[middle] < key) {
start = middle + 1;
} else {
end = middle - 1;
}
}
return -1;
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
所查找元素在數組中的下標為:9
1
2
2.求連續子數組的最大和?
{10,2,-1,9,-10,-99}最小值:不能超過0x80000000
從頭到尾遍歷整個數組,用一個整數記錄子數組和的最大值,如果子數組和大於這個值,則更新最大值,如果子數組和為負,子數組和清零,然後繼續往後遍歷。考慮數組全是負數、數組為空等邊界情況即可。
package mypratice;
/**
* @Package: mypratice
* @Author:kkz
* @Description:
* @Date:Created in 2018/10/16 22:45
*/
public class ConstantArray {
public static int SumOfSubArray(int[] array) {
if (array.length==0 || array==null) {
return 0;
}
int currentSum = 0; //存儲當前連續n項的和
int max = 0; //存儲連續子數組和的最大值
for (int i = 0; i < array.length; i++) {
if(currentSum<=0){ //如過當前連續n項的和小於等於0,則沒必要與後面的元素相加
currentSum = array[i]; //currentSum重新賦值
}else{
currentSum += array[i]; //如果currentSum的值大於0,則繼續與後面的元素相加,
}
if(currentSum>max){ //每次改變currentSum的值都有與max進行比較
max = currentSum; //如果currentSum的值大於max,則將currentSum的值賦值給max
}
}
return max;
}
public static void main(String[] args) {
int[] array = {10,2,-1,9,-10,-99};
int result = SumOfSubArray(array);
System.out.println("連續子數組的最大和為:"+result);
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
連續子數組的最大和為:20
1
3.交換兩個數?
package mypratice;
import java.util.Arrays;
/**
* @Package: mypratice
* @Author:kkz
* @Description:
* @Date:Created in 2018/10/16 22:24
*/
public class ChangeValue {
public static void main(String[] args) {
int[] arr = {1, 2, 3, 4, 5};
int temp = 0; //引入臨時變數,賦初始值為0
temp = arr[1]; //將arr[1]的值賦給temp temp = 1
arr[1] = arr[4]; //將arr[4]的值賦給arr[1] arr[1] = 5
arr[4] = temp; //將temp的值賦給arr[4] arr[4] = 1
System.out.println(Arrays.toString(arr)); //返回指定數組的字元串形式
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
[1, 5, 3, 4, 2]
1
4.逆置數組?{1,2,3,4}===》{4,3,2,1}
package mypratice;
import java.util.Arrays;
/**
* @Package: com.k
* @Author:kkz
* @Description:
* @Date:Created in 2018/10/14 21:09
*
* 逆置數組?{1,2,3,4}===》{4,3,2,1}
* 將數組中的
*/
public class TestArray {
public static void main(String[] args) {
int n = 1/2;
int[] array = {1,2,3,4};//聲明並初始化數組
for(int i = 0;i < (array.length)/2; i ++){
int tmp = array[i];//把數組中的值賦給臨時變數
array[i] = array[array.length - i - 1];//將後面的值賦給數組前面
array[array.length - i - 1] = tmp;//將臨時變數里的值賦給後面 即交換數組前一半與後面的元素
}
for(int i = 0;i < array.length; i ++) {}
System.out.println(Arrays.toString(array));
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
[4, 3, 2, 1]

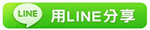
※C 中gets() 提示 warning: this program uses gets(), which is
※對Linux定時任務的認識
TAG:程序員小新人學習 |