Arduino+Processing模擬雷達掃描效果教程
一、前言
閱讀此文前請戳這個視頻觀看模擬雷達掃描視頻演示,先了解效果,有直觀感受再來看下文。
圖1 雷達掃描效果
二、硬體準備
圖2 所需硬體
如圖2所示,需要Arduino主板(最好加上擴展板)1塊、超聲波感測器1個、伺服電機(舵機)1個,1602液晶顯示屏(可選)。
我將超聲波感測器的Trig、Echo分別連接在擴展板的4、5號引腳,將伺服電機連接在擴展板的9號引腳上,為了簡化程序,後續我沒有使用液晶顯示屏,請你自行添加相關程序。
三、軟體準備
1、Arduino IDE 1.8.9
圖3 Arduino IDE
2、Processing 3.3.5
圖4 Processing
備註:
1、上述軟體版本是我自己調試成功的版本,略低版本可能會有兼容問題,請注意你的軟體版本號;
2、如果Processing的程序編輯器內不能輸入、顯示中文,請在「文件」、「偏好設定」里,選擇「啟用複雜字體輸入」並在「編輯器和控制台字體」中選擇「宋體」即可顯示或輸入中文;
圖5 修改Processing偏好設定
3、如果Processing的展示界面不能顯示中文,請在程序里裝載中文字型檔,如圖6第5、19、20行代碼所示。
圖6 裝載中文字型檔
四、編寫程序
程序分兩步實現:
第一步:使用Arduino IDE,編寫伺服電機180度循環搖頭、獲取超聲波感測器返回值(與障礙物的距離值)、向串口輸出伺服電機轉動的角度和超聲波感測器的距離值(注意要和Processing中獲取串口值的格式一致),代碼如下(請注意修改超聲波感測器、伺服電機的引腳號為你自己的實際引腳號):
#include<stdio.h>
#include <Servo.h>
Servo mServo; //創建一個舵機控制對象
//當前角度
int mAngleNum = 0;
//當前是正向旋轉還是反向旋轉
char mFront = 0;
//超聲波測距引腳
const int mTrigPin = 4;
const int mEchoPin = 5;
//當前距離
int mDistance = 0;
//像串口發送數據 發送到processing
void sendStatusToSerial();
//測距
void ranging();
void setup() {
// put your setup code here, to run once:
Serial.begin(9600);
mServo.attach(9); // 該舵機由arduino第9腳控制
pinMode(mTrigPin, OUTPUT);
// 要檢測引腳上輸入的脈衝寬度,需要先設置為輸入狀態
pinMode(mEchoPin, INPUT);
}
void loop() {
// put your main code here, to run repeatedly:
//多角度設置
mServo.write(180 - mAngleNum);
//超聲波測距
ranging();
//發數據
sendStatusToSerial();
delay(60);
if( mFront == 0 )
{
mAngleNum ++;
if( mAngleNum > 180 )
{
mFront = 1;
}
}
else
{
mAngleNum --;
if( mAngleNum < 0 )
{
mFront = 0;
}
}
}
//發送當前狀態到串口
void sendStatusToSerial()
{
char mAngleStr[6];
char mDistanceStr[6];
sprintf( mAngleStr, "%d", mAngleNum);
sprintf( mDistanceStr, "%d", mDistance);
delayMicroseconds(2);
Serial.print(mAngleStr);
Serial.print(",");
Serial.print(mDistanceStr);
Serial.print(".");
delay(50);
}
//測距
void ranging()
{
// 產生一個10us的高脈衝去觸發TrigPin
digitalWrite(mTrigPin, LOW); //低高低電平發一個短時間脈衝去TrigPin
delayMicroseconds(2);
digitalWrite(mTrigPin, HIGH);
delayMicroseconds(10);
digitalWrite(mTrigPin, LOW);
mDistance = pulseIn(mEchoPin, HIGH) / 58.0; //將回波時間換算成cm
}
第二步:使用Processing,編寫一圖7的界面、獲取串口發送過來的角度、距離值,將其轉換旋轉指針的動作、屏幕中間的紅色提示、屏幕下方的文字提示等,具體代碼如下:
圖7 模擬雷達界面圖
import processing.serial.*;
import java.awt.event.KeyEvent;
import java.io.IOException;
PFont font;
Serial myPort;
String angle="";
String distance="";
String data="";
String noObject;
float pixsDistance;
int iAngle, iDistance;
int index1=0;
int index2=0;
PFont orcFont;
void setup() {
size (1200, 700); //這個解析度自己根據你的電腦的配置和顯示屏幕配置進行更改。
smooth();
font = createFont("宋體.vlw",48);
textFont(font);
myPort = new Serial(this,"COM5", 9600); //這個串口號一定要更改。
myPort.bufferUntil(".");
}
void draw() {
fill(98,245,31);
noStroke();
fill(0,4);
rect(0, 0, width, height-height*0.065);
fill(98,245,31);
drawRadar();
drawLine();
drawObject();
drawText();
}
void serialEvent (Serial myPort) {
data = myPort.readStringUntil(".");
data = data.substring(0,data.length()-1);
index1 = data.indexOf(",");
angle= data.substring(0, index1);
distance= data.substring(index1+1, data.length());
iAngle = int(angle);
iDistance = int(distance);
}
void drawRadar() {
pushMatrix();
translate(width/2,height-height*0.074);
noFill();
strokeWeight(2);
stroke(98,245,31);
// draws the arc lines
arc(0,0,(width-width*0.0625),(width-width*0.0625),PI,TWO_PI);
arc(0,0,(width-width*0.27),(width-width*0.27),PI,TWO_PI);
arc(0,0,(width-width*0.479),(width-width*0.479),PI,TWO_PI);
arc(0,0,(width-width*0.687),(width-width*0.687),PI,TWO_PI);
// draws the angle lines
line(-width/2,0,width/2,0);
line(0,0,(-width/2)*cos(radians(30)),(-width/2)*sin(radians(30)));
line(0,0,(-width/2)*cos(radians(60)),(-width/2)*sin(radians(60)));
line(0,0,(-width/2)*cos(radians(90)),(-width/2)*sin(radians(90)));
line(0,0,(-width/2)*cos(radians(120)),(-width/2)*sin(radians(120)));
line(0,0,(-width/2)*cos(radians(150)),(-width/2)*sin(radians(150)));
line((-width/2)*cos(radians(30)),0,width/2,0);
popMatrix();
}
void drawObject() {
pushMatrix();
translate(width/2,height-height*0.074);
strokeWeight(9);
stroke(255,10,10); // red color
pixsDistance=iDistance*((height-height*0.1666)*0.025);
if(iDistance<40){
line(pixsDistance*cos(radians(iAngle)),-pixsDistance*sin(radians(iAngle)),(width-width*0.505)*cos(radians(iAngle)),-(width-width*0.505)*sin(radians(iAngle)));
}
popMatrix();
}
void drawLine() {
pushMatrix();
strokeWeight(9);
stroke(30,250,60);
translate(width/2,height-height*0.074);
line(0,0,(height-height*0.12)*cos(radians(iAngle)),-(height-height*0.12)*sin(radians(iAngle)));
popMatrix();
}
void drawText() {
pushMatrix();
if(iDistance>40) {
noObject = "檢測範圍外";
}else {
noObject = "檢測範圍內";
}
fill(0,0,0);
noStroke();
rect(0, height-height*0.0648, width, height);
fill(98,245,31);
textSize(25);
text("10cm",width-width*0.3854,height-height*0.0833);
text("20cm",width-width*0.281,height-height*0.0833);
text("30cm",width-width*0.177,height-height*0.0833);
text("40cm",width-width*0.0729,height-height*0.0833);
textSize(28);
text("對象: " + noObject, width-width*0.875, height-height*0.0277);
text("角度: " + iAngle +" °", width-width*0.48, height-height*0.0277);
text("距離: ", width-width*0.26, height-height*0.0277);
if(iDistance<40) {
text(" " + iDistance +"cm", width-width*0.225, height-height*0.0277);
}
textSize(25);
fill(98,245,60);
translate((width-width*0.4994)+width/2*cos(radians(30)),(height-height*0.0907)-width/2*sin(radians(30)));
rotate(-radians(-60));
text("30°",0,0);
resetMatrix();
translate((width-width*0.503)+width/2*cos(radians(60)),(height-height*0.0888)-width/2*sin(radians(60)));
rotate(-radians(-30));
text("60°",0,0);
resetMatrix();
translate((width-width*0.507)+width/2*cos(radians(90)),(height-height*0.0833)-width/2*sin(radians(90)));
rotate(radians(0));
text("90°",0,0);
resetMatrix();
translate(width-width*0.513+width/2*cos(radians(120)),(height-height*0.07129)-width/2*sin(radians(120)));
rotate(radians(-30));
text("120°",0,0);
resetMatrix();
translate((width-width*0.5104)+width/2*cos(radians(150)),(height-height*0.0574)-width/2*sin(radians(150)));
rotate(radians(-60));
text("150°",0,0);
popMatrix();
}
圖8 我的模仿作品
總之,實現模擬雷達掃描效果,只需要能將角度、距離值發送到串口,然後使用Processing將獲得的數據模擬出來即可,這種方法可以推而廣之,將其他感測器的返回值也發送給Processing,將返回值圖形化,說不定你也可以做出其他不一樣的創意出來。
好好學習,天天向上,初始化工作室和你一起學習Arduino知識,快去動手做吧,做出不一樣的雷達掃描效果分享給大家。

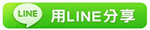